A popular refrain in object-oriented code is to favor object composition over inheritance. It offers more flexibility, less overhead, and ends up being easier to reason about. The same concept applies to functions, too!
A common pattern is to have a function that does some work and then calls another function, which does some work and calls another function, and so on. The problem is that the first function then cannot be used or tested without the entire chain of other functions it calls. This is the function equivalent of "inheritance."
Instead, we can compose functions, that is, pipe them together. Instead, take the output of the first function and pass it to the second, then take the second's output and pass it to the third, etc. That way, each of the functions can be reused, tested, and understood in isolation, then we can stick them together like LEGO blocks to build whatever series of steps we want.
That is, instead of this:
<?php
function A($in)
{
// ...
return B($out);
}
function
B($in)
{
// ...
return C($out);
}
function
C($in)
{
// ...
return $out;
}
?>
Structure it like this:
<?php
function A($in)
{
// ...
return $out;
}
function
B($in)
{
// ...
return $out;
}
function
C($in)
{
// ...
return $out;
}
function
doit($in) {
$out = A($in);
$out = B($out);
$out = C($out);
return $out;
}
?>
Now `A()`, `B()`, and `C()` are all easier to read, understand, and test, and we can more easily add a step B2 or D if we want. So powerful is this concept that many languages have a native operator for piping functions together like that. PHP doesn't, yet, but it's straightforward enough to do in user space anyway.
Want to know more about functional programming and PHP? Read the whole book on the topic: Thinking Functionally in PHP.
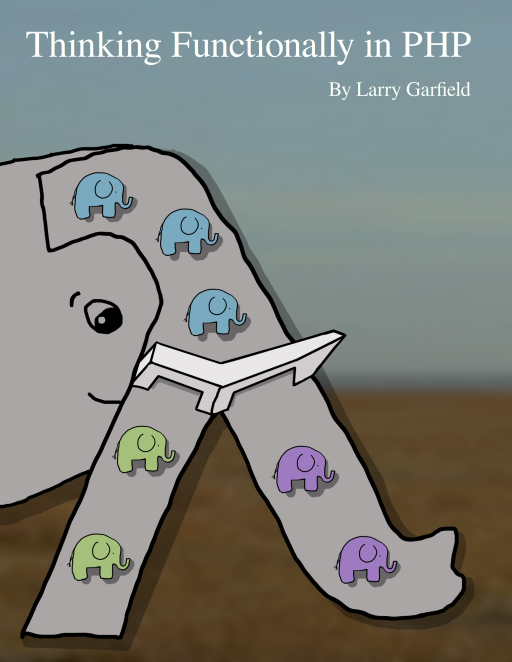