An immutable variable is one whose value doesn't change after it has been first set. PHP doesn't natively support that, but we can write our classes in such a way to simulate it. For example, rather than this:
<?php
class Point
{
private int $x;
private int $y;
public function __construct(int $x, int $y)
{
$this->x = $x;
$this->y = $y;
}
public function moveUp(int $by): void
{
$this->y += $by;
}
}
?>
We can write this:
<?php
class Point
{
private int $x;
private int $y;
public function __construct(int $x, int $y)
{
$this->x = $x;
$this->y = $y;
}
public function
moveUp(int $by): Point
{
$new = clone ($this);
$new->y += $by;
return $new;
}
}
?>
In the second version, once a given `Point` object is created it will never change. We can safely pass it to another function or method and be guaranteed that its value won't change without us knowing. Instead, any attempt to change it results in a new object, with its own identity, representing the new point in space. (In practice there would be other methods here as well, but we're focusing on just the mutation part.)
Code that uses immutable variables is easier to think about, because we don't have to worry about "does passing this object to this function change it?" We know it doesn't. Once we know something about an object we can guarantee that fact doesn't change. That can make a lot of subtle bugs impossible, which means we don't have to spend time looking for or correcting them.
Want to know more about functional programming and PHP? Read the whole book on the topic: Thinking Functionally in PHP.
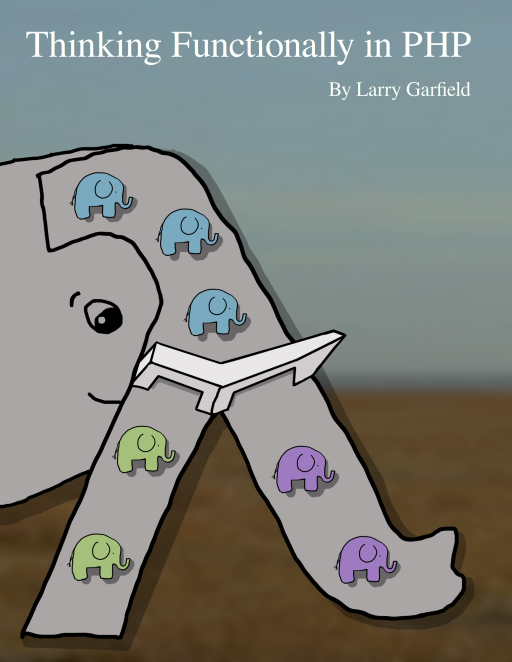