Procedural code tends to think in terms of writing out steps, and so the usual way to work with a list is to iterate it using a `for` or `foreach` loop.
Functional code tends to think in terms of the relationships and transformations between data, where those relationships and transformations are defined as functions. That means the natural way to work with a list is to define a function that is the relationship between one list and another. The most common way of doing that is with a "map," which in PHP usually means the `array_map()` function.
With `array_map()`, you give it an array and a function. You get back the result of applying that function to every element in the array, individually. Like so:
<?php
$arr = [1, 2, 3, 4, 5];
$fun = fn($x) => $x * 2;
$result = array_map($fn, $arr);
?>
The advantages of that over a `foreach` loop are:
* The function is a separate operation that can be as complex as you want.
* If it's more than a line or two, make it its own function or method somewhere and test it in isolation.
* If it's trivial, you can simply inline it.
* It's clear, visually, that every element's transformation is independent of every other's, because that's how `array_map()` works. A `foreach` loop may maintain state from one iteration to the next, but `array_map()` does not.
Want to know more about functional programming and PHP? Read the whole book on the topic: Thinking Functionally in PHP.
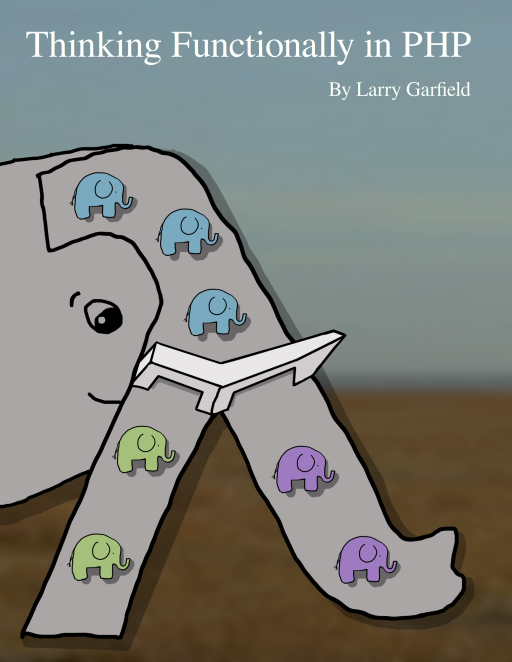