One of the many pieces of advice for a long-term sustainable code base is to keep code small. The larger a code base is, the more effort it takes to understand all the moving parts. Your brain can only keep so much mental model of your code in it at once, and if the code you're looking at is too large then what you can fit in your own "active memory" at once then you will have an increasingly hard time understanding it.
Most useful applications tend to grow larger than what the typical human can fit in their active memory, however, so you need a way to break up your code so you can load a relevant piece into your brain at once to understand and debug it. Usually that takes the form of encapsulation, coupling, cohesion, and other common object-oriented vernacular.
But what about just a pure function?
A pure function is a function that:
- has no inputs other than those explicitly specified;
- has no effect on any value other than the value it returns.
That has a number of advantages, such as being idempotent (calling it a second time with the same values is guaranteed to return the same result) and referential transparency (a function and its parameters is synonymous with its result, which can let you optimize the function away entirely in some cases). But perhaps the biggest advantage of pure functions doesn't have a fancy name: It's really easy to fit in your brain.
If you're trying to understand a given piece of code, a pure function will always be the easiest to understand because there is no need for context. There are some explicit inputs, which you can see; There is an explicit output, which you can see; And there's nothing else to care about. Reading the function (and the data definition of its parameters) is all you need to think about, because there's nothing else to think about. Every function becomes a natural "small enough to fit in your brain" unit.
A pure function will often call other pure functions, but that only creates slightly more overhead. Once you know a function is pure, it's easy to mentally "unload" as a black box that you can deal with separately. Go read that code first, then put it out of your brain and focus on the next function.
Moreover, functional programming-style code tends to favor function composition over direct function calls. That is, rather than function A calling function B which calls function C, you call function A and pass its return value to function B, then pass B's return value to C. That whole process can be wrapped up into another function if necessary. That makes it even easier to focus on only one function at a time, which is virtually guaranteed to fit in your brain at once.
Want to know more about functional programming and PHP? Read the whole book on the topic: Thinking Functionally in PHP.
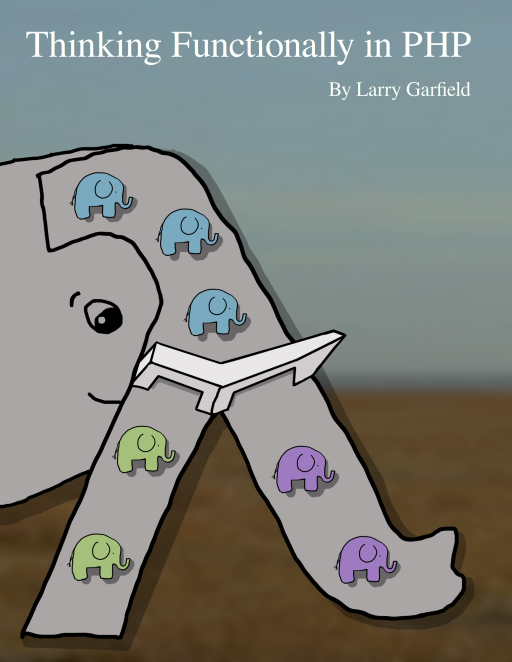